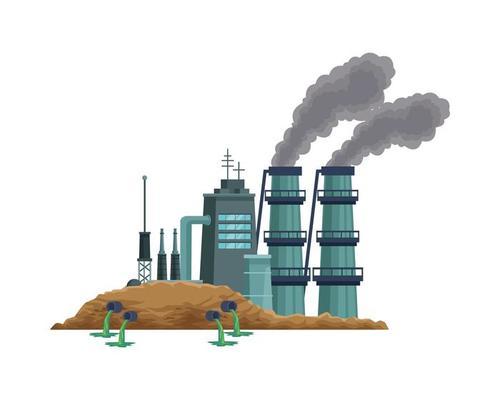
A Journey into Software Architecture Design Patterns
The art of crafting robust and maintainable code can be like writing an arrangement for a grand symphony. Just as a composer would harmonize various musical instruments to portray a certain emotion, software architects orchestrate design patterns to construct elegant and scalable software solutions. In this series, we embark on a journey into the realm of software architecture and popular design patterns. We will explore their history and why they are so popular for building resilient software systems.
Why Learn Design Patterns
Learning design patterns is essential for every aspiring software engineer and seasoned developer alike. They offer a structured approach to problem-solving, enabling developers to leverage established solutions rather than reinventing the wheel. Mastery of design patterns empowers developers to architect cleaner, more maintainable, and extensible codebases, fostering collaboration and facilitating code reuse across projects.
In this blog series we will go over the following design patterns:
-
Singleton: Ensures that a class has only one instance and provides a global point of access to that instance.
-
Factory Method: Defines an interface for creating objects, but allows subclasses to alter the type of objects that will be created.
-
Abstract Factory: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
-
Builder: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
-
Prototype: Creates new objects by copying an existing object, thus avoiding the need for subclassing.
-
Adapter: Allows incompatible interfaces to work together, acting as a bridge between two incompatible interfaces.
-
Decorator: Adds new functionality to an object dynamically without altering its structure, providing a flexible alternative to subclassing for for extending functionality.
-
Observer: Defines a one-to-many dependency between objects, ensuring that when one object changes state, all its dependents are notified and updated automatically.
-
MVC (Model-View-Controller): Separates an application into three interconnected components - Model, View, and Controller - to improve code organization and maintainability.
-
MVVM (Model-View-ViewModel): Enhances the separation of concerns in UI development by introducing a ViewModel layer, facilitating testability and flexibility in user interface design.
Join me as I dive deeper into each design pattern and unravel the inner workings of what makes an application architecture elegant and scalable. I'm excited to get started, so let's gooooo!